Your shopping cart is empty!
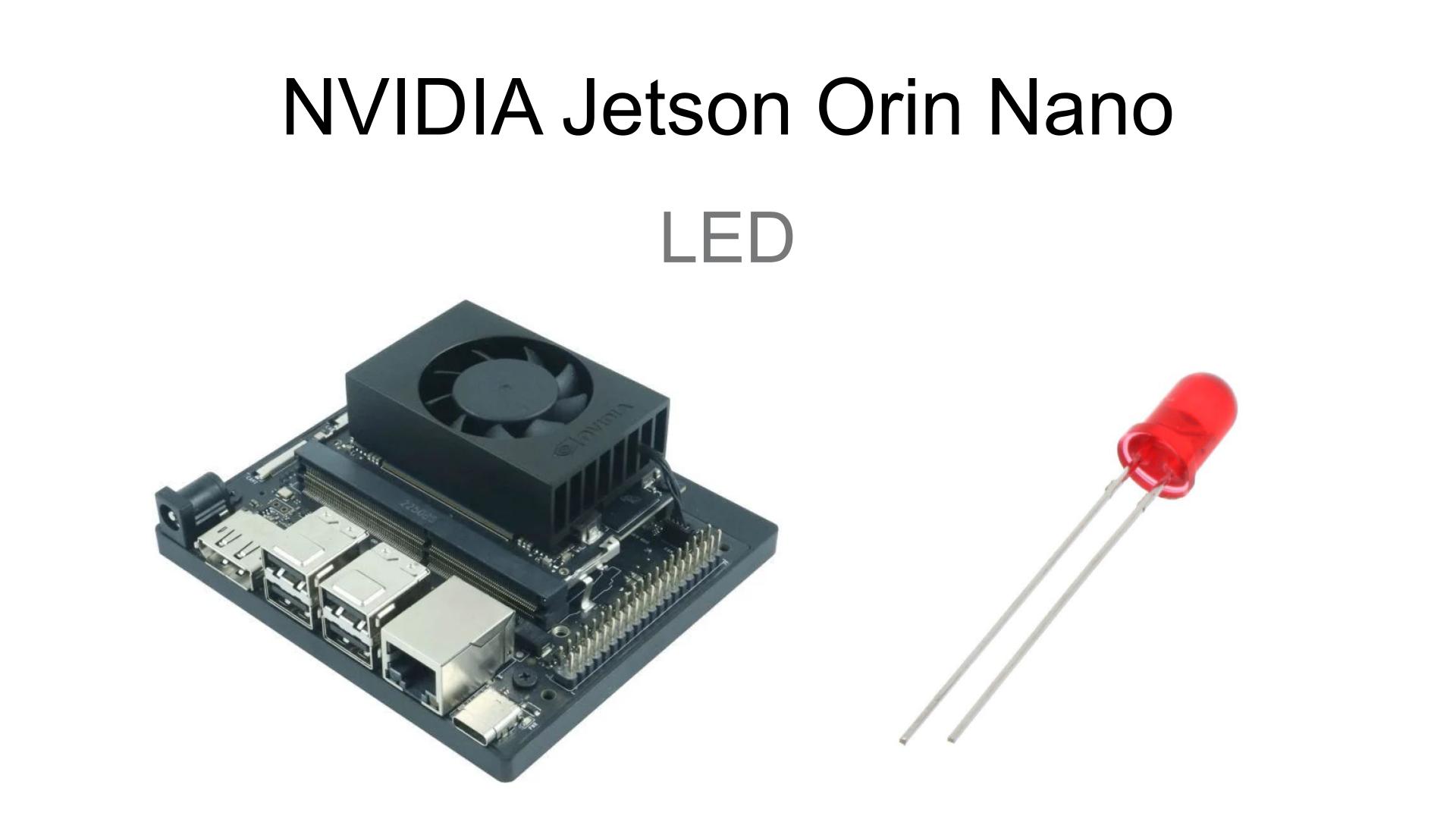
Use LED with Nvidia Jetson Orin Nano GPIO
- Huck Phin Ch’ng
- 24 Jul 2024
- Tutorial
- 297
Introduction
The NVIDIA Jetson Orin Nano Developer Kit carrier boards include a 40-pin expansion header (UART, SPI, I2S, I2C, GPIO). Much like an Arduino or Raspberry Pi, the GPIO pins can be used to toggle a simple LED on and off.
Hardware
NVidia Jetson Orin Nano 8GB Dev Kit with JetPack 5.1.2
LED
Connect the positive leg of your LED to the 7th pin on the Nvidia Jetson Orin Nano.
Connect the negative leg to a GND (ground) pin on the Nvidia Jetson Orin Nano such as the 6th pin.
Software
Turn on your NVIDIA Jetson Orin Nano, open a new terminal window, and first check that Jetson.GPIO is installed
sudo pip install Jetson.GPIO
It should say Requirement already satisfied: Jetson.GPIO in …
Create a new Python file named blinking_led.py
touch blinking_led.py
Now let’s edit the blinking_led.py file
gedit blinking_led.py
For the start we are going to import the necessary libraries needed
import Jetson.GPIO as GPIO
import time
Then we declare which PIN the LED is connected to
led_pin = 7
We then set the GPIO to use the physical pin number and for the pin to output
GPIO.setmode(GPIO.BOARD)
GPIO.setup(led_pin, GPIO.OUT, initial=GPIO.LOW)
Then start a try except block. Inside the try block, we will use a while statement. Inside the while statement, the code will repeatedly execute. So here we repeatedly set our LED to turn on, wait for 500 milliseconds, set our LED to turn off and wait for another 500 milliseconds.
try:
while True:
GPIO.output(led_pin, GPIO.HIGH) # Turn LED on
time.sleep(0.5) # Wait for 500ms
GPIO.output(led_pin, GPIO.LOW) # Turn LED off
time.sleep(0.5) # Wait for 500ms
Lastly, finish the try except block with the except block specifying KeyboardInterrupt. This block will make our program safely close when we want to close the program.
except KeyboardInterrupt:
print("Exiting gracefully")
GPIO.cleanup() # Cleanup all GPIOs
The completed code should look like this:
import Jetson.GPIO as GPIO
import time
# Pin Definitions
led_pin = 7 # The pin where the LED is connected
# Pin Setup
GPIO.setmode(GPIO.BOARD) # Use physical pin numbering
GPIO.setup(led_pin, GPIO.OUT, initial=GPIO.LOW) # Set pin as an output
try:
while True:
GPIO.output(led_pin, GPIO.HIGH) # Turn LED on
time.sleep(0.5) # Wait for 500ms
GPIO.output(led_pin, GPIO.LOW) # Turn LED off
time.sleep(0.5) # Wait for 500ms
except KeyboardInterrupt:
print("Exiting gracefully")
GPIO.cleanup() # Cleanup all GPIOs
Click on the Save button to save the code and then close gedit (the text editor program)
We can now launch the blinking_led.py program
python3 blinking_led.py
When you are finished, you can press Ctrl + C to safely stop the program
Hardware Components
NVIDIA Jetson Orin Nano 8GB Dev Kit
S$799.00 S$799.00
Nvidia Jetson Orin Nano Training Kit - AI Compu...
S$899.00 S$990.00 S$899.00